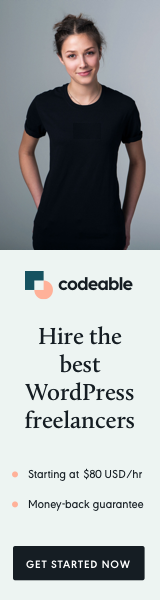
When working in WordPress, encoding data to JSON format is often necessary, especially when creating plugins, themes, or handling REST API responses. Both json_encode()
and wp_json_encode()
are commonly used for this purpose, yet each serves slightly different roles. The core PHP function json_encode()
provides basic JSON encoding, while WordPress’s wp_json_encode()
offers additional features tailored to WordPress environments. In this post, we’ll dive into the distinctions between these two functions to help you choose the most suitable one for your WordPress development needs.
Understanding json_encode()
json_encode()
is a fundamental PHP function that converts a PHP array or object into JSON format. It’s used broadly across PHP applications, including WordPress, to format data for frontend use or for API communication.
Key Characteristics of json_encode()
- Core PHP Function:
json_encode()
is available in any PHP environment, making it versatile for general use. - No Built-In Error Handling: If JSON encoding fails,
json_encode()
simply returnsfalse
, with no additional error information. This limitation can make debugging challenging. - No UTF-8 Guarantee:
json_encode()
doesn’t automatically ensure UTF-8 encoding, meaning non-UTF-8 characters could cause unexpected behavior, including partial or failed encoding.
Usage Example of json_encode()
Using json_encode()
is straightforward:
$data = ['key' => 'value'];
$json_data = json_encode($data); // Output: {"key":"value"}
While json_encode()
is simple to use, keep in mind its limitations when working in WordPress, particularly when dealing with complex data that may include non-UTF-8 characters or require custom JSON formatting.
Understanding wp_json_encode()
WordPress introduced wp_json_encode()
as a WordPress-specific wrapper for json_encode()
. This function provides enhanced error handling, UTF-8 consistency, and a filter hook for custom encoding options, making it a safer choice for WordPress developers.
Key Characteristics of wp_json_encode()
- WordPress-Specific Wrapper:
wp_json_encode()
builds onjson_encode()
to handle WordPress-specific requirements more effectively. - Error Handling: If JSON encoding fails,
wp_json_encode()
logs an error message in WordPress’s debug log, making it easier to identify and troubleshoot issues. - UTF-8 Consistency:
wp_json_encode()
ensures the output is UTF-8 compatible, preventing issues with non-UTF-8 characters that can causejson_encode()
to fail or produce inaccurate data. - Customizable JSON Options:
wp_json_encode()
includes thewp_json_encode_options
filter, allowing developers to modify JSON encoding options, such as enabling pretty print.
Usage Example of wp_json_encode()
Here’s an example of wp_json_encode()
in action:
$data = ['key' => 'value'];
$json_data = wp_json_encode($data); // Output: {"key":"value"}
Even with simple data, using wp_json_encode()
in WordPress provides additional benefits, such as error logging and UTF-8 encoding, that improve reliability and consistency across WordPress applications.
Key Differences Between json_encode() and wp_json_encode()
To help you better understand when to use each function, here’s a quick comparison of the main differences:
Feature | json_encode() | wp_json_encode() |
---|---|---|
Type | Core PHP function | WordPress-specific wrapper |
Error Handling | Returns false on failure, no debug info | Logs errors in the WordPress debug log |
UTF-8 Consistency | No | Yes |
Customizable Options | No | Yes, via the wp_json_encode_options filter |
Use Case | General PHP applications | WordPress-specific encoding needs |
Why Use wp_json_encode() in WordPress Development?
- Improved Error Handling: When
json_encode()
fails, it simply returnsfalse
, leaving developers with limited information on the cause of the failure. By contrast,wp_json_encode()
automatically logs an error message in WordPress’s debug log if encoding fails, providing helpful insights for troubleshooting. - UTF-8 Consistency: Non-UTF-8 characters can disrupt the JSON encoding process, potentially causing
json_encode()
to produce incomplete or incorrect data. Since WordPress uses UTF-8 as its standard character encoding,wp_json_encode()
ensures the output is compatible with UTF-8, making it more robust and reliable for handling user inputs and content. - Customizable JSON Options: With the
wp_json_encode_options
filter, developers can modify the encoding options to suit their needs. For example, you can enable JSON pretty print for easier readability:
add_filter('wp_json_encode_options', function($options) {
return $options | JSON_PRETTY_PRINT;
});
This filter allows for additional flexibility in encoding JSON data, making wp_json_encode()
a better choice for developers who need fine-tuned control over JSON formatting in WordPress.
Practical Examples of json_encode() and wp_json_encode() in WordPress
To better understand the scenarios where json_encode()
and wp_json_encode()
might be used, let’s look at a few examples:
Example 1: Basic JSON Encoding for Frontend Use
When sending data from the backend to the frontend in WordPress, encoding it in JSON is essential. Here’s how wp_json_encode()
can help:
$data = ['message' => 'Hello, world!', 'status' => 200];
echo wp_json_encode($data);
Using wp_json_encode()
ensures that the data will be consistently encoded and compatible with WordPress, especially when special characters or non-standard inputs are involved.
Example 2: API Response Handling
If you’re creating a custom API endpoint in WordPress, encoding the response in JSON is necessary. In this case, wp_json_encode()
is ideal due to its error logging and UTF-8 consistency:
function custom_api_endpoint() {
$response = ['status' => 'success', 'data' => ['key' => 'value']];
wp_send_json(wp_json_encode($response));
}
Here, wp_json_encode()
helps ensure that the API response is properly encoded, while wp_send_json()
takes care of setting the correct headers.
Example 3: Selective Encoding Options
Let’s say you want to enable pretty printing for debugging. With wp_json_encode()
, you can do this easily by adding a filter:
add_filter('wp_json_encode_options', function($options) {
return $options | JSON_PRETTY_PRINT;
});
$data = ['name' => 'WordPress', 'plugin' => 'My Plugin'];
echo wp_json_encode($data);
The JSON output will now include line breaks and indentation, making it easier to read during development.
Choosing Between json_encode() and wp_json_encode()
When to Use json_encode()
- For general PHP applications where WordPress-specific functionality is not required.
- If you don’t need WordPress-specific UTF-8 handling or error logging.
- In cases where specific JSON options don’t need customization.
When to Use wp_json_encode()
- For WordPress plugins and themes, where JSON output needs to align with WordPress standards.
- When handling user input or content that may contain special characters or non-UTF-8 data.
- If you require error handling and debugging information within WordPress.
- For customizing JSON options in WordPress using the
wp_json_encode_options
filter.
Final Thoughts: Best Practices for JSON Encoding in WordPress
While json_encode()
provides a straightforward solution for encoding data to JSON, wp_json_encode()
adds WordPress-specific benefits that make it the superior choice within WordPress development. By using wp_json_encode()
, you can ensure UTF-8 compatibility, enjoy enhanced error handling, and leverage custom encoding options—ultimately making your code safer, more reliable, and aligned with WordPress standards.