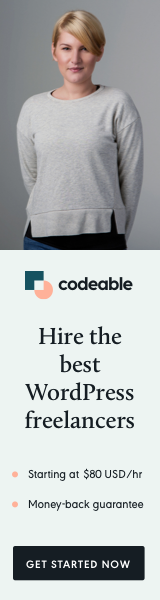
When developing a WordPress site or plugin, understanding the concept of hooks is crucial. Hooks allow developers to extend and modify the core functionality of WordPress without altering its core files. This is done through two main types of hooks: actions and filters. In this blog post, we will delve into what hooks are, how they work, and how you can use them to enhance your WordPress projects.
What Are Hooks?
Hooks in WordPress are points in the code where you can add your custom code, allowing you to modify the default behavior of WordPress. There are two types of hooks:
- Actions: These allow you to add or modify functionality at specific points in the execution of WordPress. For example, you might want to send an email when a new user registers or log data when a post is published.
- Filters: These allow you to modify existing data before it is sent to the database or displayed to the user. For instance, you could use a filter to change the title of a post before it is displayed on the front end.
Understanding and mastering these hooks is essential for creating effective WordPress plugins or themes.
Why Use Hooks?
Using hooks provides several advantages:
- Maintainability: Hooks allow you to customize WordPress without modifying core files, which means your changes won’t be overwritten during updates.
- Flexibility: You can easily add new features or modify existing ones without a complete rewrite of your code.
- Collaboration: Hooks make it easier for multiple developers to work on the same project. Since everyone can use hooks to add their functionality, it reduces conflicts.
How to Use Actions
Actions are used when you want to perform a task at a specific point in the execution of WordPress. To create an action, you use the add_action()
function, which takes two primary parameters: the name of the action and the callback function you want to execute.
Example of Using Actions
Let’s say you want to send a welcome email to users when they register. You would use the user_register
action hook, which fires when a new user is created. Here’s how you can implement it:
function send_welcome_email($user_id) {
$user_info = get_userdata($user_id);
$to = $user_info->user_email;
$subject = 'Welcome to Our Site!';
$message = 'Thank you for registering! We hope you enjoy your stay.';
wp_mail($to, $subject, $message);
}
add_action('user_register', 'send_welcome_email');
In this example, the send_welcome_email()
function is triggered every time a new user registers on the site. The function retrieves the user’s email address and sends them a welcome message.
Commonly Used Action Hooks
Here are some commonly used action hooks in WordPress:
wp_head
: Fired in the<head>
section of the theme, often used to include styles or scripts.wp_footer
: Fired just before the closing</body>
tag, useful for including scripts that should load at the end of the page.init
: Fires after WordPress has been initialized, often used to register custom post types or taxonomies.admin_init
: Runs in the admin area, useful for initializing settings or scripts.
How to Use Filters
Filters allow you to modify data before it is processed or displayed. You use the add_filter()
function to create a filter, which also takes two primary parameters: the name of the filter and the callback function.
Example of Using Filters
Imagine you want to customize the title of your posts by adding a prefix. You would use the the_title
filter, which allows you to modify the post title before it’s displayed. Here’s how to do that:
function add_prefix_to_title($title) {
return 'My Custom Prefix: ' . $title;
}
add_filter('the_title', 'add_prefix_to_title');
In this example, the add_prefix_to_title()
function adds a prefix to every post title. Whenever a title is displayed on the site, it will now include “My Custom Prefix: ” before the actual title.
Commonly Used Filter Hooks
Here are some commonly used filter hooks in WordPress:
the_content
: Used to modify the post content before it’s displayed.excerpt_length
: Allows you to change the length of the post excerpt.wp_title
: Used to modify the title of the page displayed in the browser tab.widget_title
: Modifies the title of a widget before it’s displayed.
Best Practices for Using Hooks
- Use Unique Function Names: To avoid conflicts with other plugins or themes, prefix your function names with your plugin or theme name.
- Check for Function Existence: Before adding hooks, use
function_exists()
to ensure that you don’t accidentally redeclare a function. - Keep It Organized: Group related hooks together and comment on what each hook does for better maintainability.
- Use Priorities: When adding hooks, you can set priorities to control the order in which functions are executed. Lower numbers execute first, and higher numbers execute later. For example:
add_action('init', 'my_function', 10);
- Consider Performance: Be mindful of performance when using hooks, especially in high-traffic sites. Avoid complex operations in hooks that fire frequently.
Debugging Hooks
Debugging hooks can sometimes be tricky. Here are a few tips to help:
- Use
error_log()
: You can use theerror_log()
function to log messages to the PHP error log. This is helpful for debugging your hook callbacks. - Check Hook Execution: If your action or filter isn’t working, ensure it’s being executed at the right time. You can add temporary debug messages to verify when your hooks are firing.
- Use Query Monitor: The Query Monitor plugin can help you see all the hooks that are fired on a page and can be invaluable for debugging.
Conclusion
Mastering WordPress hooks is a fundamental skill for any developer looking to create powerful and flexible plugins or themes. By understanding how to effectively use actions and filters, you can significantly enhance the functionality of your WordPress projects while maintaining clean and manageable code.
As you delve deeper into WordPress development, remember that the possibilities are vast. Hooks are a gateway to customizing and optimizing WordPress for your specific needs. Whether you’re sending welcome emails, modifying content, or creating complex functionality, hooks empower you to tailor WordPress to your vision. Start experimenting with hooks today, and unlock the full potential of your WordPress development journey!