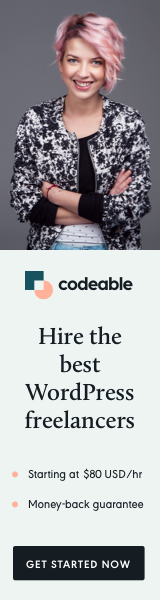
In WordPress development, managing media files efficiently is a critical task. One common challenge is avoiding duplicate entries in the media library. Whether you’re developing a theme, or plugin, or just customizing your WordPress site, it’s essential to check if a media file already exists in the database to prevent unnecessary duplication. In this blog post, I’ll share a simple yet effective function that helps you determine if a media file already has a corresponding database entry.
The Problem with Duplicate Media Entries
Duplicate media entries can lead to several issues:
- Increased Database Size: Unnecessary duplicates consume more storage space.
- Confusion in Media Management: It’s harder to manage and locate specific media files when there are duplicates.
- Potential Performance Issues: Larger databases can lead to slower query performance, especially on media-heavy sites.
The Solution: Checking for Existing Attachments
To address this, we need a function that checks whether a given media file URL corresponds to an existing attachment in the WordPress database. Here’s how the function looks:
function has_attachment( $url ) {
global $wpdb;
$url = str_replace( '', '/', $url );
if ( $wpdb->get_results( $wpdb->prepare( "SELECT ID FROM {$wpdb->posts} WHERE guid = %s", $url ) ) ) {
return true;
}
return false;
}
Understanding the Function
- The function
has_attachment
takes a media file URL as its parameter. - It uses the global
$wpdb
object to interact with the WordPress database. - The URL is sanitized to ensure the correct format (replacing backslashes with forward slashes).
- A SQL query checks the
wp_posts
table for a matchingguid
(Globally Unique Identifier), which in WordPress corresponds to the media file URL. - If a matching entry is found, the function returns
true
; otherwise, it returnsfalse
.
Use Cases and Benefits
- Plugin and Theme Development: When developing themes or plugins that handle media files, this function can be used to check for existing files before attempting to upload or create new entries.
- Import Scripts: If you’re writing a script to import content into WordPress, including media files, you can use this function to avoid re-uploading files that are already present.
- Media Management Tools: For custom media management solutions, this function helps maintain a cleaner, more efficient media library.
Best Practices and Considerations
- Performance Optimization: While this function is useful, consider caching the results if you’re checking multiple URLs in one request to avoid repeated database queries.
- Database Changes: Be aware that direct database interactions might be affected by changes in WordPress core. Keep your functions updated.
- Alternative Approaches: Depending on your specific use case, alternative methods like checking attachment filenames or using WordPress media functions might be more appropriate.
Effective media management is crucial for maintaining a streamlined and efficient WordPress site. With the has_attachment
function, you can easily verify the existence of media files in your database, preventing unnecessary duplication and keeping your media library organized.
As always, when dealing with database interactions, ensure that your code is secure and optimized for the best performance.
2 replies on “Verifying Existing Attachments in the Database”
What’s the difference between this approach and the built-in function `attachment_url_to_postid()`?
I also think that `guid` does not have to be a URL at all. I’m not sure about this.
Context: When you use
wp_insert_attachment
with a relative file path, WordPress internally converts this relative path into an absolute path and stores it in the_wp_attached_file
post meta field. By storing the absolute path in the_wp_attached_file
meta field, WordPress ensures that it can accurately locate and access the attachment file when needed.So, the main difference is in the SQL query:
attachment_url_to_postid()
:$sql = $wpdb->prepare( "SELECT post_id, meta_value FROM $wpdb->postmeta WHERE meta_key = '_wp_attached_file' AND meta_value = %s", $path );
custom function:
$sql = $wpdb->prepare( "SELECT ID FROM {$wpdb->posts} WHERE guid = %s", $url );
Then the
_wp_attached_file
has the full path and relative path for this we need to check the posts table where we have the guid.So, to conclude the
attachment_url_to_postid()
has better efficiency & ease of use, but it doesn’t cover 100% of the cases so it’s reliability is less that the custom function.