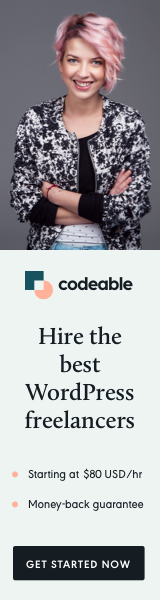
Quality assurance (QA) is an integral part of WordPress plugin development. It ensures your plugin functions as intended, provides a seamless user experience, and adheres to security standards. This guide dives into actionable QA methods, complete with code snippets and practical tools.
1. Code Quality Assurance
Objective: Ensure your code is clean, maintainable, and adheres to WordPress coding standards.
a. Code Review
Method: Use GitHub Pull Requests or GitLab Merge Requests for peer reviews. Here’s how to set up a pre-commit hook with PHPCS to enforce coding standards:
# Install WordPress coding standards
composer global require "wp-coding-standards/wpcs"
# Add this to your pre-commit hook
#!/bin/sh
phpcs --standard=WordPress --ignore=vendor .
b. Static Code Analysis
Tool: Use PHPCS for static analysis. Here’s how to analyze your plugin:
# Analyze code
phpcs --standard=WordPress path/to/your/plugin
c. Unit Testing
Method: Write PHPUnit tests to validate individual components. Below is an example test for a simple function:
// Example function
function add_numbers($a, $b) {
return $a + $b;
}
// PHPUnit Test
class ExampleTest extends WP_UnitTestCase {
public function test_add_numbers() {
$this->assertEquals(4, add_numbers(2, 2));
}
}
Run the tests with:
phpunit --bootstrap=path/to/your/plugin/bootstrap.php path/to/your/tests
2. User Experience (UX) Quality Assurance
Objective: Ensure the plugin is easy to use and visually consistent.
a. Usability Testing
Method: Create a simple survey or use a tool like Google Forms to collect user feedback.
Example Survey Questions:
- How easy was it to install and activate the plugin?
- Were the plugin settings intuitive to configure?
b. UI Testing
Method: Use Cypress for automated UI tests. Here’s an example test:
describe('Plugin UI Test', () => {
it('Checks the settings page loads', () => {
cy.visit('/wp-admin/admin.php?page=plugin-settings');
cy.contains('Plugin Settings');
});
});
Run the test with:
npx cypress open
3. Functional Quality Assurance
Objective: Ensure all features work as expected.
a. Functional Testing
Method: Use Behat for behavior-driven testing. Example Behat test:
Feature: Search and Replace
Scenario: Replace text in Elementor widget
Given I am logged in as an administrator
And I am on the "Elementor Widgets" page
When I replace "old text" with "new text"
Then I should see "new text" in the widget preview
Run with:
vendor/bin/behat
b. Integration Testing
Method: Use WP CLI for automated integration tests. Example command:
# Check plugin compatibility
wp plugin install classic-editor --activate
wp plugin install plugin-to-test --activate
4. Performance Quality Assurance
Objective: Ensure the plugin performs efficiently and scales well.
a. Performance Testing
Tool: Query Monitor can help analyze performance.
Installation:
wp plugin install query-monitor --activate
Usage: After activation, go to the WordPress admin bar and access Query Monitor to analyze page load times and database queries.
5. Security Quality Assurance
Objective: Ensure the plugin is secure against common vulnerabilities.
a. Security Testing
Tool: Use WPScan to identify security issues.
# Scan your WordPress installation
wpscan --url https://example.com --api-token YOUR_API_TOKEN
Code Example for Nonces:
function my_plugin_form() {
?>
<form method="post">
<?php wp_nonce_field('my_plugin_action', 'my_plugin_nonce'); ?>
<input type="text" name="example_field">
<input type="submit" value="Submit">
</form>
<?php
}
function my_plugin_save() {
if (!isset($_POST['my_plugin_nonce']) || !wp_verify_nonce($_POST['my_plugin_nonce'], 'my_plugin_action')) {
wp_die('Nonce verification failed!');
}
// Save data securely
}
6. Compliance and Documentation
Objective: Maintain up-to-date and comprehensive documentation.
a. Documentation Review
Method: Use tools like MkDocs for structured documentation.
Example Configuration (mkdocs.yml
):
site_name: My Plugin Docs
nav:
- Home: index.md
- Installation: installation.md
- Usage: usage.md
- API Reference: api.md
Generate and serve the documentation with:
mkdocs serve
7. Regression Testing
Objective: Ensure changes do not break existing functionality.
a. Regression Testing
Method: Use PHPUnit and Behat tests to ensure all existing tests pass after new changes. Example PHPUnit test:
// Test a critical functionality
public function test_critical_functionality() {
$this->assertTrue(function_to_test());
}
Run all tests with:
phpunit && vendor/bin/behat
8. Release and Post-Release Testing
Objective: Ensure the plugin is stable before and after release.
A. Pre-Release Testing
Method: Deploy the plugin to a staging environment for final checks.
# Deploy to staging
scp -r path/to/plugin user@staging-server:/path/to/wp-content/plugins/
B. Post-Release Monitoring
Method: Use Sentry for error monitoring.
Installation:
composer require sentry/sdk
Usage:
Sentryinit(['dsn' => 'https://examplePublicKey@o0.ingest.sentry.io/0']);
function problematic_function() {
SentrycaptureException(new Exception('Something went wrong'));
}
Monitor the dashboard for real-time error tracking.
Conclusion
Quality assurance is essential in WordPress plugin development to ensure high performance, security, and user satisfaction. By systematically applying the methods and tools discussed, you can deliver a superior plugin that stands out in the competitive WordPress ecosystem. Whether it’s through rigorous code reviews, usability tests, or performance monitoring, integrating QA practices at every stage of development guarantees a robust and reliable plugin.