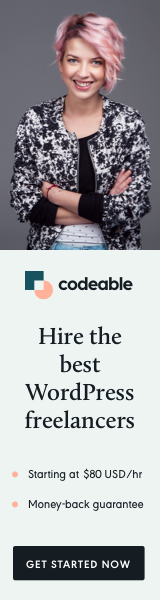
Creating custom blocks in WordPress’s Block Editor (Gutenberg) offers a high degree of flexibility and control over your content. In this post, we’ll dive into how you can implement custom headings with different sizes (H1 to H6) using registerBlockType
from WordPress’s block editor and the RichText
component.
Defining Block Attributes
Firstly, we need to define our block attributes. We will need two attributes: contentHeading
for the heading text and contentHeadingSize
for the heading size (ranging from H1 to H6).
contentHeading: {
type: 'string',
source: 'html',
selector: '.custom-heading',
default: ''
},
contentHeadingSize: {
type: 'string',
enum: ['h1', 'h2', 'h3', 'h4', 'h5', 'h6'],
default: 'h2',
},
Adding Controls in the Editor
To allow users to select the heading size, we use a SelectControl
within a PanelBody
. This control offers a dropdown menu for choosing between H1 to H6.
<PanelBody title={ __( 'Heading', 'textdomain' ) } initialOpen={ false }>
<SelectControl
label={ __( 'Size', 'textdomain' ) }
help={ __( 'Select heading size.', 'textdomain' ) }
value={ attributes.contentHeadingSize}
onChange={ ( newSize ) => setAttributes( { contentHeadingSize: newSize } ) }
options={ [
{ label: __( 'Heading 1', 'textdomain' ), value: 'h1' },
{ label: __( 'Heading 2', 'textdomain' ), value: 'h2' },
// ... other options
] }
/>
</PanelBody>
Editing Function
In the edit function, we use the RichText
component to allow users to input and edit the heading text. The tagName
attribute dynamically sets the heading size based on the selected option.
edit: function( { attributes, setAttributes } ) {
return(
<div { ...useBlockProps() }>
<RichText
tagName={ attributes.contentHeadingSize }
className='custom-heading'
value={ attributes.contentHeading }
onChange={ ( newHeading ) => setAttributes( { contentHeading: newHeading } ) }
placeholder="Enter heading here..."
/>
</div>
);
},
Save Function
In the save function, we use RichText.Content
to output the heading tag. The tagName
and className
attributes ensure that the correct heading size and styles are applied.
save: function( { attributes } ) {
return(
<div { ...useBlockProps.save() }>
{ attributes.contentHeading && (
<RichText.Content
tagName={ attributes.contentHeadingSize }
className='inblocks-custom-heading'
value={ attributes.contentHeading }
/>
)}
</div>
);
},
Conclusion
By integrating these elements into your custom block, you can easily offer a rich text editor experience in the Block Editor, complete with customizable heading sizes. This enhances the flexibility and user-friendliness of your custom blocks, allowing for dynamic and versatile content creation.