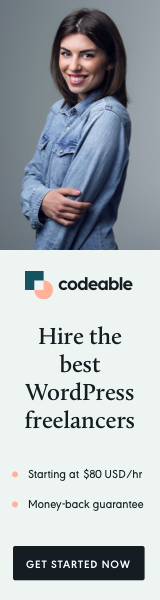
Adding a dark and light mode toggle in WordPress is a great way to enhance user experience. It lets users choose their preferred theme, providing flexibility and accessibility. In this tutorial, we’ll use a combination of PHP, CSS, and JavaScript to create a toggle button and apply theme changes dynamically.
What Is a Dark and Light Mode Toggle?
A dark and light mode toggle is a switch that allows users to alternate between dark and light themes. This feature is increasingly popular due to its benefits, including improved readability and reduced eye strain in low-light conditions.
By incorporating this toggle in your WordPress menu, you ensure that users can quickly access the option without searching for settings.
Code to Add a Theme Toggle Button to WordPress
Below is the code you need to implement this feature. It’s designed to work with any WordPress theme and menu.
PHP Code: Add the Toggle Button
The following code adds a toggle button to your WordPress navigation menu dynamically:
function generic_theme_toggle_button($items, $args) {
if ($args->theme_location) { // Check if a theme location exists
$toggle_button = '
<li class="menu-item theme-toggle">
<div class="theme-switch-wrapper">
<label class="theme-switch">
<input type="checkbox" id="theme-toggle" />
<span class="slider round"></span>
</label>
</div>
</li>';
$items .= $toggle_button;
}
return $items;
}
add_filter('wp_nav_menu_items', 'generic_theme_toggle_button', 10, 2);
This code hooks into the wp_nav_menu_items
filter to add the toggle button at the end of your menu. You can customize the placement by editing the $items
variable.
CSS Code: Define Theme Variables
Use CSS custom properties to define color schemes for dark and light modes:
body {
--accent: #87ceeb;
--accent-alt: #3b5863;
--contrast: #ffffff;
--contrast-2: #f7f8f9;
--contrast-3: #f0f0f0;
--base: #b2b2be;
--base-2: #575760;
--base-3: #222222;
--base-4: #242628;
--base-5: #202020;
}
body.light-mode {
--accent: #87ceeb;
--accent-alt: #3b5863;
--contrast: #222222;
--contrast-2: #333333;
--contrast-3: #575760;
--base: #f5f5f5;
--base-2: #e7e7ea;
--base-3: #ffffff;
--base-4: #f7f8f9;
--base-5: #ededf2;
}
These variables allow you to create distinct color palettes for both modes.
JavaScript Code: Implement Toggle Functionality
The JavaScript below handles the theme switching and saves the user’s preference in localStorage
:
document.addEventListener("DOMContentLoaded", function () {
const toggles = document.querySelectorAll("#theme-toggle");
const body = document.body;
// Set theme based on saved preference
const savedTheme = localStorage.getItem("generic-theme");
if (savedTheme) {
const isLightMode = savedTheme === "light";
body.classList.toggle("light-mode", isLightMode);
toggles.forEach((toggle) => (toggle.checked = isLightMode));
}
// Toggle theme on switch
toggles.forEach((toggle) => {
toggle.addEventListener("change", function () {
const isLightMode = toggle.checked;
body.classList.toggle("light-mode", isLightMode);
localStorage.setItem("generic-theme", isLightMode ? "light" : "dark");
});
});
});
This script ensures the toggle works seamlessly across page loads, preserving the user’s preference.
How the Code Works
- PHP Code: Adds the toggle button to any navigation menu assigned to a theme location.
- CSS Variables: Define a flexible color palette for dark and light modes.
- JavaScript Logic: Handles switching the theme and persists the selection using
localStorage
.
Benefits of Adding a Theme Toggle
- Enhanced User Experience: Users can choose their preferred mode for better readability.
- Accessibility: Improves usability for those sensitive to light.
- Modern Design: Gives your site a polished, interactive touch.
Customizing the Code
You can adjust the code to match your specific needs:
- Button Placement: Modify the
$items
variable in the PHP code to position the toggle button anywhere in your menu. - Color Palette: Update the CSS variables to match your site’s branding.
- Default Theme: Set the default theme in the JavaScript by changing the
body.classList
logic.
Testing Your Implementation
After adding the code to your theme, test the following:
- Toggle button appearance and functionality.
- Correct application of dark and light modes.
- Local storage saving and retrieving the user’s choice.
Conclusion
Adding a dark and light mode toggle in WordPress menus is a simple yet impactful feature. By following this guide, you can implement a generic solution that works across themes and provides a better experience for your visitors.