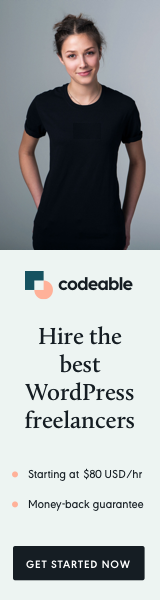
Why Your WooCommerce Store Needs an Account Deletion Feature
In today’s digital landscape, giving users control over their personal data isn’t just good practice—it’s increasingly becoming a legal requirement. With regulations like GDPR in Europe and CCPA in California, offering customers a straightforward way to delete their accounts is essential for any e-commerce store.
WooCommerce, while powerful, doesn’t include a built-in account deletion feature in its core functionality. This gap leaves many store owners wondering how to implement this critical privacy feature without compromising their order history or business analytics.
In this comprehensive guide, we’ll walk through implementing a secure, user-friendly account deletion system for your WooCommerce store that:
- Gives customers control over their data
- Maintains your order history for accounting purposes
- Follows privacy best practices
- Provides valuable feedback on why customers leave
Understanding WooCommerce Account Deletion Challenges
Before diving into the code, it’s important to understand the unique challenges of implementing account deletion in WooCommerce:
- Order History Preservation: Completely deleting user accounts could remove valuable order history needed for accounting and tax purposes.
- Data Privacy Balance: You need to respect user privacy while maintaining necessary business records.
- User Experience: The deletion process should be straightforward but include confirmation steps to prevent accidental deletions.
- Feedback Collection: Understanding why customers leave provides valuable business insights.
Our solution addresses all these concerns with a thoughtful implementation that respects both customer privacy and business needs.
Step-by-Step Implementation Guide
Step 1: Add the Delete Account Button to the Account Details Page
The first step is adding a clear, accessible deletion option to the standard WooCommerce account page. We’ll place this at the bottom of the account details form with appropriate warning text:
// Add delete account button to Account Details page
function custom_slug_add_delete_account_button() {
?>
<fieldset>
<br /><hr />
<p class="woocommerce-form-row woocommerce-form-row--wide form-row form-row-wide">
<label for="delete_account"><?php esc_html_e( 'Delete your account', 'woocommerce' ); ?></label>
<span class="woocommerce-delete-account-info"><?php esc_html_e( 'Once you delete your account, there is no going back. Please be certain.', 'woocommerce' ); ?></span>
</p>
<p class="woocommerce-form-row form-row">
<button type="button" class="woocommerce-Button button" id="show-delete-account-modal" style="border: 0; background-color: #e2401c; color: #fff; margin-top: 0">
<?php esc_html_e( 'Delete My Account', 'woocommerce' ); ?>
</button>
</p>
</fieldset>
This adds a bold, red “Delete My Account” button with a clear warning message to set appropriate expectations for users.
Step 2: Create a Confirmation Modal
To prevent accidental deletions, we’ve created a modal dialog that requires users to confirm their decision by entering their password and optionally providing feedback:
<!-- Delete Account Modal -->
<div id="delete-account-modal" style="display: none; position: fixed; z-index: 9999; left: 0; top: 0; width: 100%; height: 100%; overflow: auto; background-color: rgba(0,0,0,0.4); padding-top: 60px;">
<div style="background-color: #fefefe; margin: 5% auto; padding: 20px 20px 5px 20px; border: 1px solid #888; width: 80%; max-width: 500px;">
<span class="close" style="color: #aaa; float: right; font-size: 28px; font-weight: bold; cursor: pointer;">×</span>
<h3><?php esc_html_e( 'Confirm Account Deletion', 'woocommerce' ); ?></h3>
<p><?php esc_html_e( 'Are you sure you want to delete your account? This action cannot be undone and all your account data will be permanently removed.', 'woocommerce' ); ?></p>
<form method="post" class="woocommerce-delete-account-form">
<?php wp_nonce_field( 'woocommerce-delete-account', 'woocommerce-delete-account-nonce' ); ?>
<p class="form-row">
<label for="delete-account-password"><?php esc_html_e( 'Enter your password to confirm', 'woocommerce' ); ?> <span class="required">*</span></label>
<input type="password" class="woocommerce-Input woocommerce-Input--text input-text" name="delete_account_password" id="delete-account-password" required />
</p>
<p class="form-row">
<label for="delete-account-reason"><?php esc_html_e( 'Reason for leaving (optional)', 'woocommerce' ); ?></label>
<textarea name="delete_account_reason" id="delete-account-reason" rows="3"></textarea>
</p>
<p class="woocommerce-delete-account-buttons">
<button type="button" class="button cancel-delete" style="margin-right: 10px;"><?php esc_html_e( 'Cancel', 'woocommerce' ); ?></button>
<button type="submit" class="woocommerce-Button button" name="delete_account" value="Delete Account" style="background-color: #e2401c; color: #fff;"><?php esc_html_e( 'Delete My Account', 'woocommerce' ); ?></button>
</p>
</form>
</div>
</div>
This modal includes:
- A clear warning about the permanence of account deletion
- Password verification for security
- An optional feedback field to gather insights
- Clear cancel and confirm buttons
Step 3: Add the JavaScript for Modal Interaction
Next, we need to make the modal interactive with some simple jQuery:
<script type="text/javascript">
jQuery(document).ready(function($) {
// Show the modal when the delete account button is clicked
$('#show-delete-account-modal').on('click', function() {
$('#delete-account-modal').show();
});
// Hide the modal when the close button or cancel is clicked
$('.close, .cancel-delete').on('click', function() {
$('#delete-account-modal').hide();
});
// Also hide the modal when clicking outside of it
$(window).on('click', function(event) {
if ($(event.target).is('#delete-account-modal')) {
$('#delete-account-modal').hide();
}
});
});
</script>
This JavaScript:
- Shows the modal when the delete button is clicked
- Hides it when cancel, close, or outside the modal is clicked
- Follows standard UX patterns for modal dialogs
Step 4: Add Custom Styling
We want the feature to blend seamlessly with WooCommerce’s existing design, so we add some minimal styling:
// Add custom styling
function custom_slug_delete_account_styles() {
if ( is_account_page() ) {
?>
<style type="text/css">
.woocommerce-delete-account-info {
display: block;
margin-bottom: 10px;
color: #777;
}
#delete-account-modal h3 {
margin-top: 0;
}
.woocommerce-delete-account-buttons {
margin-top: 20px;
text-align: right;
}
</style>
<?php
}
}
add_action( 'wp_head', 'custom_slug_delete_account_styles' );
By conditionally loading these styles only on account pages, we maintain site performance while ensuring our feature looks professional.
Step 5: Process the Account Deletion
Now for the most critical part—the function that actually processes the account deletion request:
// Process account deletion
function custom_slug_process_delete_account() {
if ( isset( $_POST['delete_account'] ) ) {
// Verify nonce
if ( ! isset( $_POST['woocommerce-delete-account-nonce'] )
|| ! wp_verify_nonce( $_POST['woocommerce-delete-account-nonce'], 'woocommerce-delete-account' ) ) {
wc_add_notice( __( 'We were unable to process your request. Please try again.', 'woocommerce' ), 'error' );
return;
}
// Verify password
$current_user = wp_get_current_user();
if ( ! isset( $_POST['delete_account_password'] )
|| ! wp_check_password( $_POST['delete_account_password'], $current_user->user_pass, $current_user->ID ) ) {
wc_add_notice( __( 'Invalid password. Please try again.', 'woocommerce' ), 'error' );
return;
}
// Store feedback if provided
if ( ! empty( $_POST['delete_account_reason'] ) ) {
// Optionally save the feedback to a log or send an email
$feedback = sanitize_textarea_field( $_POST['delete_account_reason'] );
// Example: Log to a custom table or send admin email
$admin_email = get_option( 'admin_email' );
$subject = sprintf( __( 'Account Deletion Feedback from %s', 'woocommerce' ), $current_user->user_email );
$message = sprintf( __( 'User %1$s has deleted their account with the following feedback: %2$s', 'woocommerce' ), $current_user->user_email, $feedback );
wp_mail( $admin_email, $subject, $message );
}
// Process account deletion
require_once( ABSPATH . 'wp-admin/includes/user.php' );
// Get all orders for this customer
$customer_orders = wc_get_orders(
array(
'customer_id' => $current_user->ID,
'limit' => -1,
)
);
// Anonymize orders instead of deleting them
if ( ! empty( $customer_orders ) ) {
foreach ( $customer_orders as $order ) {
// Remove personal data but keep order records
$order->set_customer_id( 0 );
$order->set_billing_email( 'deleted@user.invalid' );
$order->set_billing_first_name( 'Deleted' );
$order->set_billing_last_name( 'User' );
$order->set_billing_company( '' );
$order->set_billing_address_1( 'Address Removed' );
$order->set_billing_address_2( '' );
$order->set_billing_city( 'City Removed' );
$order->set_billing_postcode( 'Removed' );
$order->set_billing_country( '' );
$order->set_billing_state( '' );
$order->set_billing_phone( '' );
// Also anonymize shipping info if needed
$order->set_shipping_first_name( 'Deleted' );
$order->set_shipping_last_name( 'User' );
$order->set_shipping_company( '' );
$order->set_shipping_address_1( 'Address Removed' );
$order->set_shipping_address_2( '' );
$order->set_shipping_city( 'City Removed' );
$order->set_shipping_postcode( 'Removed' );
$order->set_shipping_country( '' );
$order->set_shipping_state( '' );
// Save the anonymized order
$order->save();
}
}
// Delete user and reassign posts to admin (user ID 1)
if ( wp_delete_user( $current_user->ID, 1 ) ) {
// Log the user out
wp_logout();
// Redirect to home page with success message
wp_safe_redirect( add_query_arg( 'account_deleted', 'true', home_url() ) );
exit;
} else {
wc_add_notice( __( 'We were unable to delete your account. Please contact support.', 'woocommerce' ), 'error' );
}
}
}
add_action( 'template_redirect', 'custom_slug_process_delete_account' );
This function:
- Verifies the security nonce to prevent CSRF attacks
- Confirms the user’s password for authentication
- Collects and emails any feedback provided
- Crucially, anonymizes order data rather than deleting it
- Deletes the user account and logs them out
- Redirects to a confirmation page
Step 6: Display Success Message
Finally, we want to confirm to the user that their account was successfully deleted:
// Show success message after account deletion
function custom_slug_account_deleted_message() {
if ( isset( $_GET['account_deleted'] ) && 'true' === $_GET['account_deleted'] ) {
wc_print_notice( __( 'Your account has been successfully deleted. We\'re sorry to see you go!', 'woocommerce' ), 'success' );
}
}
add_action( 'woocommerce_before_shop_loop', 'custom_slug_account_deleted_message' );
add_action( 'woocommerce_before_single_product', 'custom_slug_account_deleted_message' );
add_action( 'woocommerce_before_cart', 'custom_slug_account_deleted_message' );
add_action( 'woocommerce_before_checkout_form', 'custom_slug_account_deleted_message' );
add_action( 'wp_body_open', 'custom_slug_account_deleted_message' );
By adding hooks to multiple locations, we ensure the user sees the confirmation message regardless of where they land after being redirected.
Privacy and Legal Considerations for WooCommerce Account Deletion
While implementing this feature, it’s important to consider legal compliance:
GDPR Compliance
Under GDPR, users have the “right to be forgotten,” which this feature supports. However, GDPR also acknowledges that businesses may need to retain certain information for legal reasons, such as tax records.
Our implementation strikes this balance by:
- Completely removing personal identification from orders
- Preserving the order data itself for business records
- Giving clear notice to users about what will happen
Order History Management
The code preserves order history but anonymizes personal information by:
- Setting a generic “Deleted User” name
- Removing addresses, phone numbers, and other personal details
- Disconnecting the order from any user account
This approach satisfies both privacy requirements and business record-keeping needs.
Benefits for Your WooCommerce Store
Implementing an account deletion feature offers several advantages:
- Legal Compliance: Meet requirements for privacy regulations worldwide
- Customer Trust: Demonstrate respect for user privacy and data control
- Valuable Feedback: Gather insights on why customers leave your store
- Clean User Database: Remove inactive accounts while preserving order data
Final Thoughts and Next Steps
Providing users with control over their data is increasingly important in today’s privacy-conscious world. This WooCommerce account deletion implementation offers a balanced approach that respects user privacy while maintaining critical business records.
For store owners looking to further enhance their privacy features, consider also implementing:
- Data export functionality
- Enhanced privacy policy pages
- Regular data cleanup processes for inactive accounts
By implementing these privacy-focused features, you not only comply with regulations but also build trust with your customers—a critical factor in e-commerce success.
FAQ About WooCommerce Account Deletion
Q: Will implementing this feature delete my order history?
A: No, this implementation preserves order data while anonymizing personal information.
Q: Is this compliant with GDPR?
A: Yes, it addresses the “right to be forgotten” while maintaining necessary business records.
Q: Will this affect my sales reports?
A: No, since order data is preserved, all reports will continue to function normally.
Q: Can I customize the feedback questions?
A: Absolutely! The feedback textarea can be modified to include specific questions or options.
Q: What happens to product reviews when an account is deleted?
A: By default, they’re reassigned to the admin account (user ID 1), but this can be customized.
Implementing a secure, user-friendly account deletion process is an important step in respecting customer privacy while maintaining your WooCommerce store’s data integrity. The code provided offers a complete solution that you can customize to fit your specific store needs.
Have you implemented account deletion on your WooCommerce store? What challenges did you face? Share your experiences in the comments below!