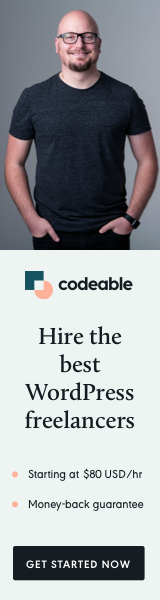
An interactive autocomplete search bar is a powerful feature that enhances user experience by providing real-time suggestions while users type. This blog post will guide you through creating a fully functional, reusable autocomplete search bar using JavaScript, CSS, and HTML.
Why Add an Autocomplete Search Bar?
Autocomplete search bars improve usability and speed by:
- Reducing typing effort with intelligent suggestions.
- Helping users find relevant content faster.
- Adding a modern, polished look to your website.
Step 1: HTML Structure
Here’s a generic structure for the autocomplete component. It includes an input field for user queries and a list for displaying suggestions.
<section class="autocomplete">
<div class="container">
<div class="autocomplete-row">
<div class="autocomplete-col">
<div id="autocomplete-animation" class="autocomplete-animation">
<input type="text" id="autocomplete-input" placeholder="" value="" autocomplete="off">
<ul id="autocomplete-suggestions" class="autocomplete-suggestions">
<li class="autocomplete-suggestions-item">Example Suggestion 1</li>
<li class="autocomplete-suggestions-item">Example Suggestion 2</li>
<li class="autocomplete-suggestions-item">Example Suggestion 3</li>
<li class="autocomplete-suggestions-item">Example Suggestion 4</li>
<li class="autocomplete-suggestions-item">Example Suggestion 5</li>
</ul>
</div>
</div>
<div class="autocomplete-col">
<div class="heading-3">
Your Search, Simplified.
</div>
<p>Explore tailored suggestions as you type. Empower your queries with dynamic search capabilities designed for user needs.</p>
</div>
</div>
<p class="text-center">
<a href="https://example.com/about-us/" class="secondary-action-button">
Learn More
</a>
</p>
</div>
</section>
This structure features:
- Input Field: Where users type their query.
- Suggestion List: Dynamically updated with relevant matches.
Step 2: CSS Styling
Here’s the CSS to style the search bar and suggestion list:
.autocomplete {
padding: 80px 0;
.heading-3 {
@media(max-width: 768px) {
margin-top: 20px;
}
}
.autocomplete-row {
margin-bottom: 80px;
@media(max-width: 768px) {
margin-bottom: 20px;
}
}
.autocomplete-col {
flex: 0 0 calc(50% - 20px);
}
.autocomplete-animation {
position: relative;
&:before {
background: url(../img/search-icon.png) no-repeat top left;
background-size: 21px 21px;
content: '';
display: inline-block;
height: 25px;
left: 15px;
position: absolute;
top: 17px;
width: 25px;
}
input[type="text"] {
background-color: $color-secondary-alt;
border: 0;
border-radius: 30px;
color: $color-primary;
margin-bottom: 20px;
padding: 15px 20px 15px 45px;
width: 100%;
&:focus {
border: 0;
box-shadow: none;
}
}
.autocomplete-suggestions {
background-color: $color-secondary-alt;
border-radius: 20px;
margin: 0;
padding: 0;
li {
align-items: center;
color: $color-primary;
cursor: pointer;
display: flex;
gap: 10px;
list-style-type: none;
padding: 10px 20px;
&:first-child {
padding-top: 20px;
}
&:last-child {
padding-bottom: 20px;
}
&:before {
background: url(../img/search-icon.png) no-repeat center;
background-size: 21px;
content: '';
display: inline-block;
height: 25px;
position: relative;
width: 25px;
}
}
}
}
}
This styling ensures the search bar is visually appealing, mobile-friendly, and easy to interact with.
Step 3: JavaScript for Autocomplete Functionality
Here’s the JavaScript to power the autocomplete feature:
document.addEventListener("DOMContentLoaded", () => {
const input = document.getElementById("autocomplete-input");
const suggestionsList = document.getElementById("autocomplete-suggestions");
let autocompleteEntriesIndex = 0;
let charIndex = 0;
let typingInterval;
let userTyping = false;
// Function to start typing the keyword
function startTyping(keyword) {
if (userTyping) return;
input.value = keyword.slice(0, charIndex + 1);
charIndex++;
const inputEvent = new Event("input");
input.dispatchEvent(inputEvent);
if (charIndex === keyword.length) {
clearInterval(typingInterval);
setTimeout(() => {
charIndex = 0;
startTypingEffect();
}, 1000);
}
}
function startTypingEffect() {
clearInterval(typingInterval);
if (autocompleteEntriesIndex === autocompleteEntries.length - 1) {
autocompleteEntriesIndex = 0;
}
const keyword = autocompleteEntries[++autocompleteEntriesIndex];
typingInterval = setInterval(() => startTyping(keyword), 50);
}
function filterSuggestions(query) {
return autocompleteEntries.filter(item => item.toLowerCase().includes(query.toLowerCase()));
}
if (input !== null) {
input.addEventListener("input", () => {
const query = input.value;
if (query.length > 0) {
const filteredSuggestions = filterSuggestions(query);
} else {
suggestionsList.classList.remove("d-block");
}
});
startTypingEffect();
}
});
This script dynamically filters the suggestions based on user input and updates the suggestion list in real time.
Benefits of This Solution
- User-Friendly: Provides real-time feedback to users as they type.
- Customizable: Easily adaptable for different use cases, such as product searches or FAQs.
- Scalable: Supports dynamic data sources, making it versatile for any website.
Conclusion
Adding an autocomplete search bar to your website is a simple yet impactful way to improve user experience. By following this guide, you can implement a fully functional, responsive, and visually appealing search bar tailored to your needs.