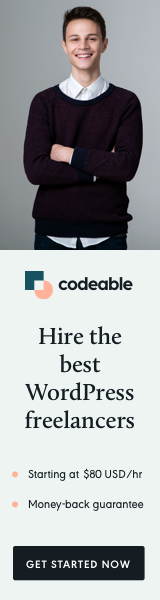
Are you looking to enhance your WordPress comment system for better user engagement? This comprehensive guide explains how to customize your WordPress comment forms using filters—specifically the powerful comment_form_defaults
filter—to create a more personalized commenting experience for your visitors.
Understanding WordPress Comment Filters
WordPress is incredibly flexible thanks to its extensive hook system. Filters, in particular, allow developers to modify data before it’s used by WordPress. Today, we’re diving deep into the comment_form_defaults
filter, which gives you precise control over your site’s comment forms.
What Are WordPress Filters?
Before we jump into customization, let’s understand what filters are. In WordPress, filters are functions that WordPress passes data through before completing an action. They allow you to modify this data rather than create it from scratch.
The filter system works by:
- WordPress generating default data
- Passing that data through any registered filter functions
- Using the modified data for its intended purpose
This approach is powerful because it preserves WordPress core functionality while allowing deep customization.
The Power of comment_form_defaults
The comment_form_defaults
filter specifically targets the default values used when generating comment forms across your WordPress site. By hooking into this filter, you can modify everything from field labels to the overall structure of your comment forms.
Let’s examine a basic implementation:
function custom_comment_login_link( $defaults ) {
$user = wp_get_current_user();
return $defaults;
}
add_filter( 'comment_form_defaults', 'custom_comment_login_link' );
This snippet creates a foundation for comment form customization. As you can see, it retrieves the current user information but doesn’t modify the defaults yet. This is where the real customization begins.
Enhancing User Experience with Comment Form Customization
Now let’s explore practical applications of this filter to improve your site’s commenting experience.
Personalizing the Comment Form for Logged-in Users
One common enhancement is creating a personalized experience for logged-in users:
function custom_comment_login_link( $defaults ) {
$user = wp_get_current_user();
if ( $user->exists() ) {
$defaults['logged_in_as'] = sprintf(
'<p class="logged-in-as">%s</p>',
sprintf(
__( 'Commenting as <strong>%1$s</strong>. <a href="%2$s">Edit your profile</a> or <a href="%3$s">log out</a>.' ),
$user->display_name,
get_edit_profile_url(),
wp_logout_url( apply_filters( 'the_permalink', get_permalink() ) )
)
);
}
return $defaults;
}
add_filter( 'comment_form_defaults', 'custom_comment_login_link' );
This enhanced version detects if a user is logged in and customizes the “Logged in as” message to be more user-friendly and informative.
Modifying Comment Form Labels and Placeholders
You can also improve usability by adjusting field labels and adding helpful placeholder text:
function custom_comment_fields( $defaults ) {
$user = wp_get_current_user();
// Customize the comment textarea field
$defaults['comment_field'] = '<p class="comment-form-comment">
<label for="comment">' . _x( 'Share your thoughts', 'noun' ) . '</label>
<textarea id="comment" name="comment" cols="45" rows="8" placeholder="What\'s on your mind about this article?" aria-required="true"></textarea>
</p>';
// Customize the comment notes before
$defaults['comment_notes_before'] = '<p class="comment-notes">
<span id="email-notes">Your email address will never be published or shared.</span>
Required fields are marked <span class="required">*</span>
</p>';
return $defaults;
}
add_filter( 'comment_form_defaults', 'custom_comment_fields' );
These modifications make your comment form more inviting and clear about what information is required and how it will be used.
Advanced Comment Form Customizations
For those looking to push the boundaries further, let’s explore some advanced techniques.
Adding Social Sharing Options
Encourage engagement by adding social sharing options to your comment form:
function custom_add_social_to_comments( $defaults ) {
$user = wp_get_current_user();
$defaults['comment_notes_after'] = '<div class="social-comment-options">
<p>After commenting, share this article:</p>
<div class="social-buttons">
<a href="https://twitter.com/intent/tweet?url=' . get_permalink() . '" class="twitter-share">Twitter</a>
<a href="https://www.facebook.com/sharer/sharer.php?u=' . get_permalink() . '" class="facebook-share">Facebook</a>
<a href="https://www.linkedin.com/shareArticle?mini=true&url=' . get_permalink() . '" class="linkedin-share">LinkedIn</a>
</div>
</div>';
return $defaults;
}
add_filter( 'comment_form_defaults', 'custom_add_social_to_comments' );
Implementing GDPR Compliance Checkbox
Ensure your comment form meets GDPR requirements by adding a consent checkbox:
function custom_gdpr_comment_checkbox( $defaults ) {
$user = wp_get_current_user();
$consent_checkbox = '<p class="comment-form-consent">
<input id="consent" name="consent" type="checkbox" value="yes" required="required" />
<label for="consent">I consent to having this website store my submitted information so they can respond to my inquiry.</label>
</p>';
$defaults['comment_notes_before'] .= $consent_checkbox;
return $defaults;
}
add_filter( 'comment_form_defaults', 'custom_gdpr_comment_checkbox' );
Optimizing Comment Forms for Better Engagement
Beyond the technical aspects, considering the user psychology behind commenting can significantly boost engagement.
Encouraging Quality Comments
Modify the comment field title to guide users toward more meaningful contributions:
function custom_encourage_quality_comments( $defaults ) {
$user = wp_get_current_user();
$defaults['title_reply'] = 'Join the Discussion';
$defaults['title_reply_to'] = 'Reply to %s';
$defaults['label_submit'] = 'Post Your Contribution';
return $defaults;
}
add_filter( 'comment_form_defaults', 'custom_encourage_quality_comments' );
These subtle wording changes can psychologically prompt users to leave more thoughtful comments.
Maintaining Performance While Customizing
While customizing your comment forms, it’s essential to keep performance in mind. Here are some best practices:
- Minimal Processing: Only perform the necessary operations inside your filter function.
- Efficient Code: Avoid nested loops or complex calculations.
- Caching: Consider caching any data that doesn’t change frequently.
For example, an efficiency-focused implementation might look like:
function custom_efficient_comment_mods( $defaults ) {
static $user_status = null;
// Cache user status check
if ( $user_status === null ) {
$user = wp_get_current_user();
$user_status = $user->exists();
}
if ( $user_status ) {
// Modifications for logged-in users
$defaults['logged_in_as'] = '...';
} else {
// Modifications for guests
$defaults['must_log_in'] = '...';
}
return $defaults;
}
add_filter( 'comment_form_defaults', 'custom_efficient_comment_mods' );
Conclusion: Creating a Unique Commenting Experience
Customizing WordPress comment forms through the comment_form_defaults
filter provides a powerful way to enhance user engagement on your site. By personalizing the comment experience, you can encourage more visitors to join the conversation and build a stronger community around your content.
Remember that the most effective customizations balance aesthetics, usability, and technical performance. Start with small changes, test them thoroughly, and gradually implement more advanced features as you become comfortable with the filter system.
Have you implemented custom comment forms on your WordPress site? What challenges did you face? Share your experiences in the comments below!