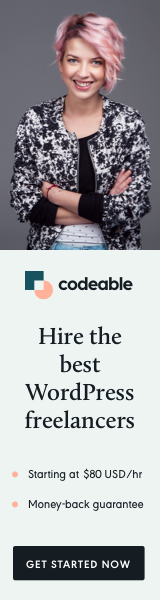
In the quick tip below, I will show you one method of how to load and use your WordPress theme or plugin style options on the front-end.
First, let’s assume you have already registered some settings using the register_setting
and add_settings_field
function in the backend.
For example, you want to have primary
and secondary
colors your users can change in the backend, and at the same time, you want to apply them in the front-end of your theme or plugin.
You want to somehow use those user-selected options in the front-end without breaking the WordPress standard way of loading styles with wp_enqueue_style
.
The CSS
The solution would be to load the settings in the header.php
before wp_head
function and then assign them as global variables using the :root {}
selector. (Note: Assigning default values is optional.)
<?php
$uuid_primary_color = ( isset( get_option( 'uuid-primary-color' ) ) ) ? get_option( 'uuid-primary-color' ) : '#2277cc' ;
$uuid_secondary_color = ( isset( get_option( 'uuid-primary-color' ) ) ) ? get_option( 'uuid-secondary-color' ) : '#323436';
?>
<style>
:root {
--uuid-primary-color: <?php echo $uuid_primary_color; ?>;
--uuid-secondary-color: <?php echo $uuid_secondary_color; ?>;
}
</style>
Now you can easily use the set variables in your stylesheet file using var(--uuid-primary-color)
or var(--uuid-secondary-color)
Note: Be sure to add a unique prefix to your variables since other plugin and theme developers use the :root{}
selector for global variables.
The SASS
You can do the same if you are using SASS and SCSS files.
For example, you have _variables.scss
a file defining all your global variables.
Then, instead of having something like $primary_color: #2277cc;
you replace it with $primary_color: var(--uuid-primary-color)
.
After that, you can follow your standard workflow and use the $primary_color
throughout your SCSS files.
You can use this method with logos, icons, and even some text by using the content
with :before
and :after
pseudo selectors.
When Using a Plugin
Well, if you are working with a plugin and you cannot modify your HTML head which should be handled by the active theme and loaded with get_header
You need to load the :root{}
selector using the wp_add_inline_style
function.
function load_user_options() {
$uuid_primary_color = ( isset( get_option( 'uuid-primary-color' ) ) ) ? get_option( 'uuid-primary-color' ) : '#2277cc' ;
$uuid_secondary_color = ( isset( get_option( 'uuid-primary-color' ) ) ) ? get_option( 'uuid-secondary-color' ) : '#323436';
$css = ":root {
--uuid-primary-color: $uuid_primary_color;
--uuid-secondary-color: $uuid_secondary_color;
";
wp_add_inline_style( 'your-main-css-handle', $css );
}
The inline style will be loaded after your main stylesheet, but since we are using only the root{}
selector, you don’t have to worry that your variables are accessible.
‘Til the next time.