Table of Contents [hide]
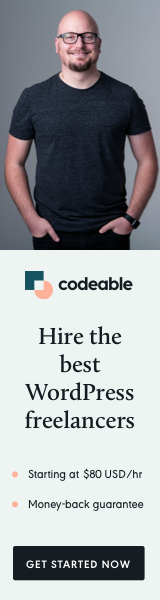
Introduction
In this tutorial, we’ll walk through the steps to add a light/dark theme toggle to your website using the GeneratePress theme. This feature enhances user experience by allowing visitors to switch between themes according to their preferences.
Prerequisites
- Basic knowledge of JavaScript and CSS.
- A WordPress website with the GeneratePress theme installed.
Step 1: Understanding the Code
First, let’s break down the provided JavaScript and CSS code.
JavaScript Code Explanation
UUID.themes
: This object holds the color schemes for dark and light themes.UUID.applyTheme()
: A function to apply the selected theme by changing CSS variables.UUID.toggleLogo()
: Changes the website logo depending on the theme.- Initial Theme Setup: Checks for saved themes or system preferences and applies them.
- Theme Switch Event Listener: Toggles the theme when the switch is clicked.
CSS Code Explanation
.theme-switch-wrapper
: Styles the theme switch container..theme-switch
: Hides the checkbox and styles the slider.- Transition Effects: Adds smooth transitions when the theme is switched.
Step 2: Adding the Toggle HTML Code
- Open your WordPress dashboard.
- Navigate to Appearance > Theme Editor.
- Select the
functions.php
file. - Paste the JavaScript code at the end of the file.
- Click Update File.
<?php/**
* Add the toggle button the primary main menu for WordPress.
*/
function uuid_add_theme_switch_toggle( $items, $args ) {
if ( $args->theme_location === 'primary' ) {
$items .= '<div id="theme-switch" class="theme-switch-wrapper">
<label for="theme-switch-checkbox" class="theme-switch">
<input type="checkbox" id="theme-switch-checkbox" name="theme-switch-checkbox" />
<div class="slider round"></div>
</label>
</div>';
}
return $items;
}
add_filter( 'wp_nav_menu_items', 'uuid_add_theme_switch_toggle', 10, 2 );
Step 3: Integrating the CSS
- In the Theme Editor, select the
style.css
file. - Add the provided CSS code for the theme switch.
- Update the file.
Step 4: Adding the Toggle Button
- The provided PHP function
uuid_add_theme_switch_toggle()
adds the toggle button to the primary menu. - Add this function to your
functions.php
file.
Step 5: Testing
- Visit your website and ensure the toggle appears in the primary menu.
- Test the toggle to switch between light and dark themes.
- If you already have a building site and have used only the Block editor to build your site you have used var(–color-name) in your custom stylesheet files then you won’t need to make many changes to make the dark/light theme look good.
Conclusion
Congratulations! You’ve successfully added a theme toggle to your GeneratePress website. This feature not only improves aesthetics but also enhances accessibility and user experience.
Troubleshooting
If you encounter issues:
- Check for any syntax errors in the code.
- Ensure that the JavaScript and CSS codes are correctly placed.
- Clear your browser cache to see the changes.