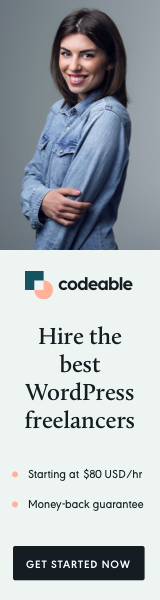
Integrating React JS into the WordPress Block Editor enhances the user experience by leveraging the power and flexibility of React. This blog post will guide you through setting up and completing a WordPress React JS environment for the Block Editor using Gulp and NPM.
Prerequisites
- Familiarity with JavaScript, ReactJS, and WordPress.
- Node.js and NPM are installed on your machine.
- A local or server setup of WordPress.
Step 1: Initializing Your Project
Start by creating a new directory for your project. Inside this directory, initialize a new NPM project by running:
npm init -y
This command will create a package.json
file that will manage all your dependencies.
Step 2: Installing Dependencies
Install the necessary packages using NPM. You’ll need Gulp for task automation, Browserify for bundling, Babelify for JSX transpilation, and other utilities:
npm install --save-dev gulp browserify babelify vinyl-source-stream vinyl-buffer gulp-uglify gulp-notify
Step 3: Setting Up Gulp
Create a gulpfile.js
in your project root and add the following code:
const gulp = require('gulp');
const browserify = require('browserify');
const babelify = require('babelify');
const source = require('vinyl-source-stream');
const buffer = require('vinyl-buffer');
const jsUglify = require('gulp-uglify');
const notify = require('gulp-notify');
const scriptsDestination = './path/to/your/destination/directory';
function scripts() {
return browserify({
entries: ['./path/to/your/main-js-file.js'],
debug: true,
transform: [babelify.configure({
presets: ["@babel/preset-env", "@babel/preset-react"]
})]
})
.bundle()
.on('error', function(err){
console.error(err);
this.emit('end');
})
.pipe(source('bundle.min.js'))
.pipe(buffer())
.pipe(jsUglify())
.pipe(gulp.dest(scriptsDestination))
.pipe(notify({ message: 'Task: "scripts" Completed 100%.', onLast: true }));
}
exports.scripts = scripts;
This script configures Gulp to use Browserify and Babelify for compiling and bundling your React JS code.
Step 4: Compiling Your JavaScript
In your gulpfile.js
, the scripts
function handles the compilation and bundling of your React JS code. Run this Gulp task by executing:
gulp scripts
This will create a minified JavaScript bundle in the specified destination directory.
Step 5: Enqueuing the Script in WordPress
To use your React JS bundle in the Block Editor, enqueue it in your WordPress theme or plugin:
function enqueue_block_editor_assets() { wp_enqueue_script( 'my-block-editor-script', get_template_directory_uri() . '/path/to/bundle.min.js', array('wp-blocks', 'wp-i18n', 'wp-element' ), null, true); } add_action( 'enqueue_block_editor_assets', 'enqueue_block_editor_assets' );
// OR if you want to target the editor content only use: //add_action( 'enqueue_block_editor', 'enqueue_block_editor_assets' );
Step 6: Creating Custom Blocks
Now that you have React JS integrated, you can start creating custom blocks for the WordPress Block Editor. Your main JavaScript file (specified in the Gulp script) will contain your block registration and React components.
Step 7: Testing and Iteration
Test your custom blocks in the WordPress Block Editor. Ensure that they load correctly and function as intended. Iterate on your code and re-run the Gulp task to recompile as needed.
Conclusion
By following these steps, you’ve successfully set up a WordPress environment using React JS for the Block Editor. This setup allows for more advanced, interactive, and dynamic block types, enhancing the content creation experience in WordPress.
Remember, the key to successful integration is understanding both WordPress and React JS. As you get more comfortable, you can expand your React JS blocks to include more complex features and interactions.