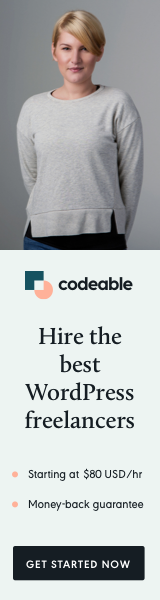
WordPress is renowned for its user-friendliness, but as your website grows in traffic and content, so do the demands on your server. Caching is one of the most powerful tools to optimize WordPress, making your site faster and more efficient by reducing database queries and server processing time. In this article, we’ll dive into three essential caching functions: wp_cache_get()
, wp_cache_set()
, and wp_cache_delete()
, exploring their use and how they can transform your WordPress site’s performance.
What is Caching in WordPress?
In the simplest terms, caching stores frequently requested data in temporary storage, or “cache,” so future requests for the same data can be served faster without repeating complex processes. In WordPress, you might cache data from database queries, external API calls, or complex calculations. The WordPress Object Cache lets you store this data temporarily so your site can respond faster.
Why Use wp_cache_get()
and wp_cache_set()
?
WordPress comes with a built-in caching API that leverages the Object Cache to store data. This internal cache, which is transient by default, is extremely useful because:
- Reduces database load: By retrieving data from cache, you save time and resources otherwise spent on database queries.
- Speeds up page load times: Serving data from cache is faster than querying the database.
- Improves user experience: Faster websites lead to better SEO rankings and happier users.
Using caching strategically can make your WordPress site not just faster but also more scalable and capable of handling high-traffic events.
How wp_cache_get()
, wp_cache_set()
, and wp_cache_delete()
Work
Let’s explore these three core functions, which provide an efficient way to manage cached data.
1. wp_cache_get( $key, $group = '' )
This function retrieves data from the cache. Here’s the syntax:
$data = wp_cache_get( 'cache_key', 'cache_group' );
$key
: A unique identifier for the data you want to retrieve.$group
: Optional. Groups related cache entries, making it easier to clear or manage multiple entries together.
Example Usage:
Imagine you have a custom function to get recent posts, which is called every time a page loads. By caching the result of this function, you can reduce database queries for repeated requests.
function get_recent_posts_cached() {
$cache_key = 'recent_posts';
$cache_group = 'posts';
// Attempt to get cached data
$recent_posts = wp_cache_get( $cache_key, $cache_group );
if ( false === $recent_posts ) {
// No cache found, retrieve data from the database
$recent_posts = get_posts( [
'numberposts' => 5,
'post_status' => 'publish',
]);
// Store data in cache
wp_cache_set( $cache_key, $recent_posts, $cache_group, 3600 ); // Cache for 1 hour
}
return $recent_posts;
}
With this setup, your site will only call the database for recent posts when the cache has expired.
2. wp_cache_set( $key, $data, $group = '', $expire = 0 )
The wp_cache_set()
function stores data in the cache with a unique key. Here’s the syntax:
wp_cache_set( 'cache_key', $data, 'cache_group', $expiration_time_in_seconds );
$key
: The identifier used to retrieve the cached data.$data
: The data to cache.$group
: Optional. Used to organize cache entries, especially useful for managing and clearing.$expire
: Optional. Expiration time for the cached data in seconds. If omitted or set to0
, the data is cached until manually cleared.
Example Usage:
Imagine you’re running a WooCommerce site and want to cache the total number of products sold to avoid repeated database queries.
function get_total_sales_cached() {
$cache_key = 'total_sales';
$cache_group = 'sales_data';
// Check if the cached data exists
$total_sales = wp_cache_get( $cache_key, $cache_group );
if ( false === $total_sales ) {
// No cache found, calculate and set cache
$total_sales = wc_get_total_sales();
wp_cache_set( $cache_key, $total_sales, $cache_group, 3600 ); // Cache for 1 hour
}
return $total_sales;
}
This approach ensures that the total sales figure is updated hourly without placing strain on your database.
3. wp_cache_delete( $key, $group = '' )
When data needs to be refreshed, you can use wp_cache_delete()
to clear it from the cache. Here’s the syntax:
wp_cache_delete( 'cache_key', 'cache_group' );
$key
: The unique identifier of the cached data to delete.$group
: Optional. The cache group where the data is stored.
Example Usage:
If a product is added, updated, or removed, you may need to clear cached data to ensure users always see the latest information. For instance:
function clear_total_sales_cache() {
$cache_key = 'total_sales';
$cache_group = 'sales_data';
// Clear the cached data
wp_cache_delete( $cache_key, $cache_group );
}
You could hook this function into WooCommerce actions like woocommerce_product_set_stock
to clear the total sales cache whenever inventory changes.
A Practical Example: Using Caching to Speed Up a Custom Query
Suppose you have a custom WordPress query that retrieves posts based on specific metadata. Here’s how to cache the query results for one hour:
function get_featured_posts() {
$cache_key = 'featured_posts';
$cache_group = 'custom_queries';
// Check if cached data exists
$featured_posts = wp_cache_get( $cache_key, $cache_group );
if ( false === $featured_posts ) {
// No cache found, execute the query
$args = [
'post_type' => 'post',
'meta_key' => 'is_featured',
'meta_value' => '1',
'posts_per_page' => 5,
];
$featured_posts = get_posts( $args );
// Set the cache with expiration
wp_cache_set( $cache_key, $featured_posts, $cache_group, 3600 );
}
return $featured_posts;
}
With this setup, your site only makes the query when the cache is expired, reducing server load and ensuring fast load times.
When Should You Use Object Caching?
- Heavy database queries: Cache data-intensive queries to serve data faster.
- External API requests: Caching API responses reduces API request frequency and speeds up load times.
- Non-changing data: Information that doesn’t frequently change is ideal for caching.
Managing Cache Groups for Clear Organization
WordPress lets you organize caches into groups. For example, you could group all post-related caches under 'posts'
or API-related caches under 'api_responses'
. Using groups helps you target specific caches to clear or manage without affecting other data.
Potential Challenges and Best Practices
While caching is incredibly powerful, improper caching can lead to outdated content or inconsistent data. Here are a few best practices:
- Cache with an expiration time: Avoid caching indefinitely unless you’re caching data that is truly static.
- Clear cache when data updates: If cached data changes, clear the cache to ensure users see updated content.
- Use unique cache keys: Avoid overlapping keys by using descriptive, unique cache keys, especially when storing different types of data.
- Group related caches: This makes managing and clearing caches easier and more targeted.
Conclusion
By implementing wp_cache_get()
, wp_cache_set()
, and wp_cache_delete()
, you can unlock a new level of speed and efficiency for your WordPress site. Not only does caching save database resources, but it also enhances user experience with faster page load times. As you apply caching to your WordPress code, remember to use meaningful cache keys, organized groups, and strategically refresh cached data when needed. With a well-planned caching strategy, you’ll be ready to handle any traffic surge your site encounters!