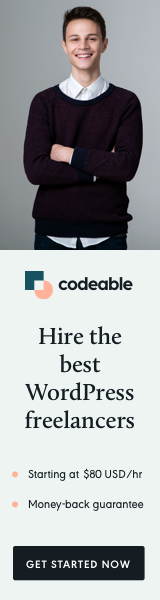
In today’s fast-paced web development landscape, creating dynamic, interactive applications has become essential. The WordPress REST API empowers developers to build modern plugins that can seamlessly integrate with other applications, platforms, and services. In this blog post, we’ll explore what the WordPress REST API is, how it works, and how you can use it to create feature-rich plugins that enhance the user experience on your WordPress site.
Understanding the WordPress REST API
The WordPress REST API provides a standardized way to interact with WordPress data using HTTP requests. This allows developers to create, read, update, and delete (CRUD) data across WordPress sites and external applications. The REST API transforms WordPress into a headless content management system (CMS), enabling developers to create applications that are separate from the traditional WordPress front-end.
Key Features of the WordPress REST API
- Access to WordPress Data: The REST API exposes various WordPress data types, including posts, pages, users, comments, and custom post types. This allows you to access and manipulate data from any external application.
- JSON Format: The API uses JSON (JavaScript Object Notation) for data interchange, making it lightweight and easy to work with in modern JavaScript frameworks and libraries.
- HTTP Methods: The REST API utilizes standard HTTP methods (GET, POST, PUT, DELETE) for operations, making it familiar for web developers.
- Authentication: The API supports various authentication methods, including cookie authentication, OAuth, and application passwords, providing secure access to protected endpoints.
Setting Up Your Development Environment
Before diving into plugin development with the REST API, you’ll need a suitable development environment. Here’s a simple setup:
- Local WordPress Installation: Use tools like Local by Flywheel, MAMP, or XAMPP to set up a local WordPress environment for testing.
- Code Editor: Choose a code editor like Visual Studio Code or Sublime Text for writing your plugin code.
- Browser Developer Tools: Familiarize yourself with the browser’s developer tools (usually F12) for inspecting network requests and debugging.
Creating a Basic Plugin
Now that you have your environment ready, let’s create a simple WordPress plugin that utilizes the REST API to fetch and display posts dynamically.
Step 1: Create Your Plugin Structure
Create a new directory in the wp-content/plugins
folder named my-rest-api-plugin
. Inside this directory, create a PHP file named my-rest-api-plugin.php
.
<?php
/**
* Plugin Name: My REST API Plugin
* Description: A simple plugin to demonstrate the WordPress REST API.
* Version: 1.0
* Author: Your Name
*/
// Exit if accessed directly
if (!defined('ABSPATH')) {
exit;
}
// Enqueue the JavaScript file
function my_rest_api_enqueue_scripts() {
wp_enqueue_script('my-rest-api-script', plugin_dir_url(__FILE__) . 'js/my-rest-api.js', array('jquery'), null, true);
wp_localize_script('my-rest-api-script', 'myRestApi', array('restUrl' => esc_url(rest_url())));
}
add_action('wp_enqueue_scripts', 'my_rest_api_enqueue_scripts');
Step 2: Create the JavaScript File
In your plugin directory, create a folder named js
and a file named my-rest-api.js
inside it. This file will handle the API requests.
jQuery(document).ready(function ($) {
// Fetch posts from the REST API
$.get(myRestApi.restUrl + 'wp/v2/posts', function (data) {
// Loop through the posts and display them
$.each(data, function (index, post) {
$('#posts').append('<h2>' + post.title.rendered + '</h2>');
$('#posts').append('<div>' + post.excerpt.rendered + '</div>');
});
});
});
Step 3: Create a Shortcode to Display Posts
Add a shortcode to your plugin that outputs a container for the posts:
// Shortcode to display posts
function my_rest_api_shortcode() {
return '<div id="posts"></div>';
}
add_shortcode('my_rest_api_posts', 'my_rest_api_shortcode');
Step 4: Activate Your Plugin
- Go to your WordPress admin dashboard.
- Navigate to Plugins and activate My REST API Plugin.
- Create a new post or page and add the shortcode
[my_rest_api_posts]
.
When you view the post or page, it should dynamically fetch and display the latest posts using the WordPress REST API.
Expanding Your Plugin with Custom Endpoints
Now that you have a basic setup, let’s extend your plugin by creating a custom REST API endpoint. This allows you to expose additional functionality tailored to your needs.
Step 1: Register a Custom Endpoint
Add the following code to your plugin file to register a custom endpoint that returns a list of custom post types.
function my_rest_api_custom_endpoint() {
register_rest_route('myplugin/v1', '/custom-posts', array(
'methods' => 'GET',
'callback' => 'my_rest_api_get_custom_posts',
));
}
add_action('rest_api_init', 'my_rest_api_custom_endpoint');
function my_rest_api_get_custom_posts() {
$args = array(
'post_type' => 'your_custom_post_type',
'post_status' => 'publish',
'numberposts' => -1,
);
$posts = get_posts($args);
return $posts;
}
Step 2: Modify the JavaScript to Use the Custom Endpoint
In my-rest-api.js
, modify the AJAX request to fetch data from your new endpoint:
// Fetch custom posts from the custom endpoint
$.get(myRestApi.restUrl + 'myplugin/v1/custom-posts', function (data) {
// Loop through the custom posts and display them
$.each(data, function (index, post) {
$('#custom-posts').append('<h2>' + post.title.rendered + '</h2>');
$('#custom-posts').append('<div>' + post.excerpt.rendered + '</div>');
});
});
Step 3: Update the Shortcode
Update your shortcode function to create a new container for displaying custom posts:
// Shortcode to display custom posts
function my_rest_api_custom_shortcode() {
return '<div id="custom-posts"></div>';
}
add_shortcode('my_rest_api_custom_posts', 'my_rest_api_custom_shortcode');
Final Thoughts
The WordPress REST API opens up a world of possibilities for plugin developers, allowing for dynamic, modern applications that enhance the user experience. By leveraging the REST API, you can create plugins that communicate with external services, fetch data asynchronously, and offer users seamless interactions.
As you continue to explore the capabilities of the REST API, consider integrating additional features such as authentication, error handling, and custom settings for your plugin. By adopting a user-centric approach and focusing on performance, you can create powerful tools that take full advantage of the dynamic web.
The key takeaway is to embrace the modern development practices that the WordPress REST API enables. With its extensive capabilities and ease of use, you can elevate your plugin development to new heights, ultimately delivering a better experience for your users and contributing to the thriving WordPress ecosystem.