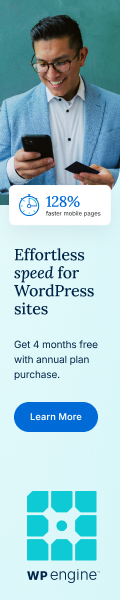
If you’ve been diving into WordPress plugin or theme development, you may have come across the functions stripslashes
and wp_unslash
. Both play a role in cleaning up slashes () from strings or arrays, often necessary for handling user input, escaping issues, and sanitizing data. However, these functions aren’t interchangeable and are optimized for different scenarios. Knowing the difference can save you from unexpected formatting issues and help you keep your code aligned with WordPress standards.
In this pro tip, we’ll explore the differences between stripslashes
and wp_unslash
and when to use each one.
Understanding stripslashes
stripslashes
is a built-in PHP function that removes backslashes from a string. This is particularly helpful when handling strings that PHP, MySQL, or other applications automatically escape with backslashes, such as user inputs or database strings. It’s straightforward to use but has limitations for complex data structures.
Example:
$name = "O\'Reilly";
$clean_name = stripslashes($name); // Output: "O'Reilly"
When to Use stripslashes
:
- General PHP use: It’s useful in PHP scripts outside of WordPress.
- Single strings only: Use it when working with simple strings, not arrays.
- Non-WordPress contexts: When you’re handling data outside of WordPress functions.
Introducing wp_unslash
WordPress adds an extra layer with its own function: wp_unslash
. This is a wrapper for stripslashes
but is more versatile, particularly when dealing with complex data structures like arrays. wp_unslash
is optimized for WordPress and recursively applies stripslashes
to each item in an array. This makes it ideal when dealing with WordPress-specific data handling, especially since inputs in WordPress are often multi-dimensional.
Example:
$data = array(
"name" => "O\'Reilly",
"bio" => "Developer\'s guide"
);
$clean_data = wp_unslash($data);
// Output: array("name" => "O'Reilly", "bio" => "Developer's guide")
When to Use wp_unslash
:
- WordPress development: This function is more in line with WordPress coding standards.
- Arrays or multi-dimensional data: Perfect for handling complex data structures like arrays from forms.
- Sanitizing user inputs and outputs: Essential for cleaning data received from forms or user-generated content.
Why You Should Prefer wp_unslash
in WordPress
WordPress has its own unique data-handling processes. It applies slashes to data automatically to avoid conflicts, like those that could arise with database queries. Therefore, wp_unslash
is the better choice in WordPress because it aligns with the framework’s internal functions, handling strings and arrays with ease.
For example, let’s say you are developing a plugin where users submit multi-field forms. Some fields could include single quotes or backslashes, especially for fields with names or textual data. If you use stripslashes
directly, you’d have to apply it to each field individually. But with wp_unslash
, you can simply pass the entire array, making it easier to manage your data.
Practical Tips for WordPress Developers
- Always use
wp_unslash
in WordPress, especially if you’re handling data that could include arrays. - Sanitize your data after using
wp_unslash
, especially for outputs directly displayed on your website. - Remember escaping and unescaping: Data from the database often has slashes, so consider
wp_unslash
when retrieving or processing such data. - Test your data handling to ensure that your application consistently displays data without unintended slashes or errors.
Conclusion
Choosing wp_unslash
over stripslashes
when developing with WordPress is more than just a stylistic choice; it’s about maintaining code consistency with WordPress standards and ensuring better handling of arrays and complex data. While stripslashes
is still useful in general PHP contexts, WordPress’s wp_unslash
simplifies data sanitization and handling, especially for plugin and theme developers who frequently work with user inputs or complex data structures.
Happy coding, and keep your data clean and slash-free!