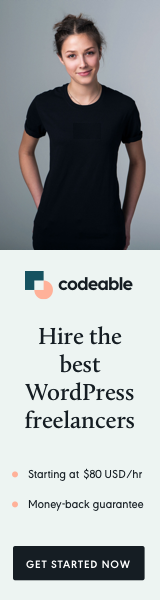
WordPress is a powerful platform for building websites, but with great power comes great responsibility, especially when it comes to security. One essential security measure in WordPress development is nonce verification. Nonces, short for “number used once,” are unique tokens generated for each user session to prevent unauthorized access or tampering of form data and links. In this quick pro tip, we’ll explore how to create and check nonces for forms and links in WordPress.
Creating Nonces
When creating a form in WordPress, you should always include a nonce field. Here’s how you can generate and include a nonce field in your form:
<form method="post">
<!-- Other form fields -->
<?php wp_nonce_field( 'my_action', 'my_nonce_field' ); ?>
<button type="submit">Submit</button>
</form>
In this example, 'my_action'
is the nonce action and 'my_nonce_field'
is the nonce field name. Replace these with appropriate values for your use case.
When creating links that perform actions in WordPress, it’s crucial to include a nonce parameter. You can generate and include a nonce in your link like this:
$url = add_query_arg( array( 'action' => 'my_action', '_wpnonce' => wp_create_nonce( 'my_action_nonce' ) ), admin_url( 'admin-post.php' ) );
echo '<a href="' . esc_url( $url ) . '">My Link</a>';
Here, 'my_action'
is the action name, and 'my_action_nonce'
is the nonce action name. Adjust these values as needed.
Verifying Nonces
Once you’ve included nonces in your forms and links, you need to verify them before processing the submitted data or performing actions. Here’s how to verify nonces:
In your form submission handler, you can verify the nonce like this:
if ( isset( $_POST['my_nonce_field'] ) && wp_verify_nonce( $_POST['my_nonce_field'], 'my_action' ) ) {
// Nonce is valid, process form data
} else {
// Nonce verification failed, handle accordingly
}
For links that trigger actions, you can verify the nonce in the action hook like this
add_action( 'admin_post_my_action', 'my_action_callback' );
function my_action_callback() {
if ( isset( $_REQUEST['_wpnonce'] ) && wp_verify_nonce( $_REQUEST['_wpnonce'], 'my_action_nonce' ) ) {
// Nonce is valid, perform action
} else {
// Nonce verification failed, handle accordingly
}
}
Implementing nonce verification for forms and links is a fundamental step in ensuring the security of your WordPress site. By following these quick pro tips, you can enhance the protection against CSRF (Cross-Site Request Forgery) attacks and unauthorized access to sensitive actions. Always remember to include nonces in your forms and links and verify them before processing any data or performing actions. Your site’s security is worth the extra effort!