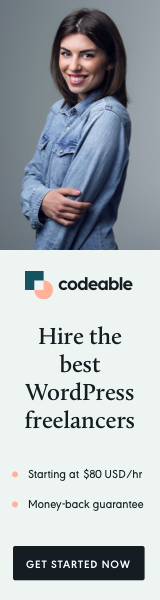
The Problem
I needed to restrict access to a single Administrator user for a plugin of mine.
Usually, if you use manage_options
when adding a new admin.php page for your plugin will restrict the access to the new pages to Admin users only (Super Admins if you are on a multisite). However, how about if you have a plugin where you also want to restrict access to the Admin users’ pages. There are many cases where WordPress sites have multiple admin access users.
The Solution
There are two steps you need to solve the problem.
The first one is to add a true/false checkbox available when you Add a New User / Edit & Update an existing one (with Admin capabilities).
And the second is to use this user meta option for your plugin page. (I am not going to discuss this in the article, but once you can make the options in the user meta table, you can easily use them to restrict access to the plugin pages)
This quick tutorial will show you how to check and restrict access to some or all Administrator users for your plugin. And you will be the only one who has access to the plugin pages you have created.
The Methods
The example code will be added in Class, and there are three main methods.
The first will be the loader, where we add actions to specify the user pages on which we want our code to be accessible.
public function extend_user_profile()
{
// Add field for user access to the Plugin.
add_action('user_new_form', array($this, 'register_profile_fields')); // /wp-admin/user-new.php
add_action('edit_user_profile', array($this, 'register_profile_fields')); // /wp-admin/user-edit.php?user_id=1
// add_action('show_user_profile', array($this, 'register_profile_fields')); // /wp-admin/profile.php
// Save user access to the Plugin as usermeta.
add_action('user_register', array($this, 'save_profile_fields')); // /wp-admin/user-new.php
add_action('edit_user_profile_update', array($this, 'save_profile_fields')); // /wp-admin/user-edit.php?user_id=1
// add_action('personal_options_update', 'cp_save_profile_fields'); // /wp-admin/profile.php
}
The second one will be the HTML checkbox that will be our true/false field.
public function register_profile_fields($user)
{
/**
* We want to restrict Administrators to change the BKPC access themselves.
*
* If Admin use is created without access to the Plugin then they won't
* be able to Add, Edit, or Update others with these options.
*/
$current_user_id = get_current_user_id();
$current_user_can = get_user_meta($current_user_id, 'user_can_access_plugin_name')[0];
$update_user_can = get_user_meta($user->ID, 'user_can_access_plugin_name')[0];
if (
!current_user_can('administrator', $current_user_id)
|| $current_user_can === null) {
return false;
}
?>
<h2><br />Extend User Options</h2>
<table class="form-table">
<tr>
<th>
<label for="user_can_access_plugin_name">User can have access<br /> to Plugin?</label>
</th>
<td>
<?php if ($update_user_can !== null) : ?>
<input type="checkbox" class="regular-text" name="user_can_access_plugin_name" value="1" id="user_can_access_plugin_name" checked />
<?php else : ?>
<input type="checkbox" class="regular-text" name="user_can_access_plugin_name" value="1" id="user_can_access_plugin_name" />
<?php endif; ?>
<em>This is useful when you want to restrict access to Plugin for other Administrators.</em>
</td>
</tr>
</table>
<?php
}
And the third one will save the input from the boolean field into the user meta table.
public function save_profile_fields($update_user_id)
{
$current_user_id = get_current_user_id();
$current_user_can = get_user_meta($current_user_id, 'user_can_access_plugin_name')[0];
if (
!current_user_can('administrator', $current_user_id)
|| $current_user_can === null) {
return false;
}
update_user_meta($update_user_id, 'user_can_access_plugin_name', $_POST['user_can_access_plugin_name']);
}
The Code
The code is pretty straightforward. You only need to be careful because we not only check and pass the current user ID, but we also do the same for the user we add, edit, or update.
And the end, you can do something like the check below in the places of your plugin where you want to restrict access (be sure to do this after wp_loaded
or admin_init
actions).
if (get_user_meta(get_current_user_id(), 'user_can_access_plugin_name') !== null) {}
What’s Next
It will get a bit complicated when we are on a multisite, but I hope that you could easily extend it and create a solution for WordPress Mu with the example I gave you above.
‘Til the next one.