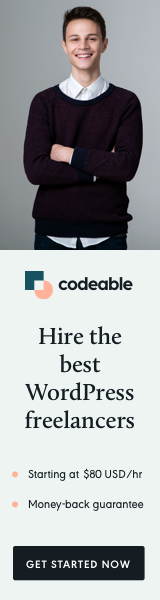
There are many different ways to set up and structure your WordPress plugin or theme CSS and JS environment files.
If you need something complex with eslint, stylelint, and PHP standards, I would recommend looking at the Composer + nodeJS method or looking into Gulp.
The method I will describe below is something I have been using and doesn’t require any 3rd party libraries.
The Requirements
You need to have:
1. PHP – https://www.php.net/downloads.php
2. Dart-SASS – https://sass-lang.com/install
Both need to have a SET_PATH to the executable files added into your global system environment paths (depending on if you are on Windows, Mac, or Linux, you need to find out how by searching on Google).
If you are using XAMPP or you already use SCSS for your CSS, then you probably have the above two already running and set up and can move on to the next step.
The Structure
You can create your own structure, but the one below is what I am using and works fine for my needs.
assets |-- build |---|-- css |---|-- js |-- css |-- js |-- scss
The files under build
will be the ones you will serve for your production site, and the other three directories js, css and scss
will be used for development.
We want the build
CSS and JS files to be minified, we can do that easily with Dart-SASS, but for JS, we need some additional code if we want to stay independent and don’t use 3rd party scripts or libraries. You will see how I do that in the run.php file.
The Development Setup
Before I talk about the files, I want to mention that I prefer to keep all my files into modules and keep the code as clean as possible, and each module methods need to have their own purpose.
For most of my projects, I use jQuery for convenience, and if you don’t be careful with larger code-based projects, things can get real messy.
JS Files
An example file structure for the JS files that are located in assets/js
.
init.js - module loader script (used for development only) utils.mjs - methods shared between the modules module1.mjs - set of methods and actions for module 1 module2.mjs - set of methods and actions for module 2 module3.mjs - set of methods and actions for module 3
And then for development, I use Ajax to load all the files (if you go DevTools and look at Network > Fetch/XHR, you can easily identify that you are running in development mode if all the modules are loading there)
Note: pluginURL can be dynamic, and all you need to do is localize it by using it. wp_localize_script()
.
CSS Files (development mode only)
To generate the development mode CSS files inside assets/css
I run a --watch
option for Dart-Sass.
This is used to save and update the CSS contents every time you change your SCSS files.
sass --watch uuid.scss ../css/uuid.css
SCSS Files
Then for the SCSS files, you don’t need to do anything special.
Just have one main file and then all the modules imported inside. These files are added into assets/scss
.
_variables.scss _base.scss _typo.scss _container.scss uuid.scss
The contents for the main SCSS file will look like this:
@import 'variables'; @import 'base'; @import 'typo'; @import 'container';
Add that’s all for development, directory, and file structure.
The Production
Now it is time to create 2 PHP scripts that will help us to generate and build our JS and CSS production files.
1. Create a run.php
This file will be inside the development assets/js
directory with the following contents.
Basically include all the module JS files, then minify the output that is saved into a variable, and at the end, print out the minified JS content.
2. Create a build.php
Next inside your build/
directory create a file with the following contents.
And you are all set up now every time you want to generate production files into your build/css
and build/js
directories just run php build.php
In the end, your file and directory structure will look like something like this:
assets |-- build |---|-- css |---|---|-- uuid.min.css |---|-- js |---|---|-- uuid.min.js |-- css |---|-- uuid.css |-- js |---|-- init.js |---|-- utils.mjs |---|-- module1.mjs |---|-- module2.mjs |---|-- module3.mjs |-- scss |---|-- _variables.scss |---|-- _base.scss |---|-- _typo.scss |---|-- _container.scss |---|-- uuid.scss
Loading Development/Production Files
To switch between development and production, you need to have a boolean variable in your WordPress theme or plugin, used to either load the production or development files.
The GitHub Repo
Check out the starter web development setup repository at GitHub.
What’s Next
The sky is the limit.
You can customize and modify the above approach and hopefully use it as a base to fit your own needs and requirements.
‘Til the next time.