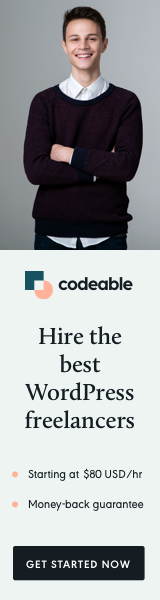
Managing a WordPress website often involves handling various media files, especially images. Whether you’re migrating a site, recovering from a data loss, or simply organizing your media files, there might be occasions when you need to rebuild and reinsert images into the WordPress Media Library from external sources. This guide will walk you through how to accomplish this, both from a URL and from a directory outside the wp-uploads folder.
Reinserting Images from a URL
Step 1: Understand the Process
Reinserting images from a URL involves downloading the image and then uploading it to your WordPress Media Library. This can be done manually or programmatically.
Step 2: Manually Download and Upload
- Navigate to the image URL in your web browser.
- Right-click on the image and choose “Save Image As” to download it to your computer.
- Go to your WordPress Dashboard, navigate to Media > Add New, and upload the downloaded image.
Step 3: Programmatically Add Images
For a more automated approach, especially useful if dealing with multiple images, you can write a PHP script to handle the process. Here’s a basic script outline:
function add_image_to_media_library_from_url( $image_url ) {
// Use WordPress built-in functions to handle the download and upload
// Make sure to include error handling and check for valid image URLs
}
Example 1: Setting a Featured Image from a Local File
Suppose you have a local image that you want to set as a featured image for a specific post. This is how you’d use the set_featured_image_from_local_file
function:
function set_featured_image_from_local_file( $post_id, $file_path ) {
// Check if the file exists
if ( ! file_exists( $file_path ) ) {
return new WP_Error( 'file_not_found', 'File not found.' );
}
// Include required files for media handling
require_once( ABSPATH . 'wp-admin/includes/image.php' );
require_once( ABSPATH . 'wp-admin/includes/file.php' );
require_once( ABSPATH . 'wp-admin/includes/media.php' );
// Prepare an array similar to that of a PHP $_FILES POST array
$file = array(
'name' => basename( $file_path ), // ex: wp-header-logo.png
'type' => mime_content_type( $file_path ), // mime type
'tmp_name' => $file_path, // path to the file
'error' => 0,
'size' => filesize( $file_path ), // file size
);
// Turn off error reporting for media_handle_sideload
$error_level = error_reporting();
error_reporting( 0 );
// Upload the file and get the attachment ID
$attachment_id = media_handle_sideload( $file, $post_id );
// Restore error reporting
error_reporting( $error_level );
// Check for handle sideload errors.
if ( is_wp_error( $attachment_id ) ) {
return $attachment_id;
}
// Set the image as the featured image
set_post_thumbnail( $post_id, $attachment_id );
}
$post_id = 123; // Replace with the ID of the post you want to modify
$local_file_path = '/path/to/your/image.jpg'; // The path to your local image file
$result = set_featured_image_from_local_file( $post_id, $local_file_path );
if ( is_wp_error( $result ) ) {
echo 'Error setting featured image: ' . $result->get_error_message();
} else {
echo 'Featured image set successfully from local file.';
}
In this example, you replace $post_id
with the ID of the post you’re targeting and $local_file_path
with the actual path to your image file.
Best Practices and Considerations
- Back-Up Your Site: Always back up your WordPress site before manipulating media files, especially when working with scripts.
- File Permissions: Ensure the directory where you’re sourcing images from and the wp-uploads folder have the appropriate permissions.
- Respect Copyright Laws: Only upload images you own or have permission to use.
- Optimize Images: To maintain website performance, optimize images for web use before uploading them to your site.
Reinserting Images from a Directory Outside wp-uploads
Step 1: Locate Your Images
Ensure you have access to the directory where your images are stored. This directory should be accessible from your WordPress server.
Step 2: Manually Upload Images
- Simply move or copy the images from the external directory to the wp-uploads folder.
- Use the WordPress Media Uploader (Media > Add New) to upload each image.
Step 3: Programmatically Move Images
If you have a large number of images, consider a PHP script to automate the process. This script can move images from the external directory to wp-uploads and then add them to the Media Library.
function add_image_to_media_library_from_directory( $file_path ) {
// Use PHP functions to move the file to wp-uploads
// Then use WordPress functions to attach the file to the Media Library
}
Example 2: Setting a Featured Image from a URL
If you want to set a featured image from an image available via a URL, you can use the set_featured_image_from_url
function:
function set_featured_image_from_url( $post_id, $image_url ) {
// Include required files for media handling
require_once( ABSPATH . 'wp-admin/includes/image.php' );
require_once( ABSPATH . 'wp-admin/includes/file.php' );
require_once( ABSPATH . 'wp-admin/includes/media.php' );
// Download the image file to a temporary location
$temp_file = download_url( $image_url );
// Check for errors
if ( is_wp_error( $temp_file ) ) {
return $temp_file;
}
// Set variables for storage and prepare for upload
$file = array();
$file['name'] = basename( $image_url );
$file['tmp_name'] = $temp_file;
// Upload the image file to WordPress
$attachment_id = media_handle_sideload( $file, $post_id );
// Check for handle sideload errors.
if ( is_wp_error( $attachment_id ) ) {
@unlink( $file['tmp_name'] ); // Clean up any temp files
return $attachment_id;
}
// Set the image as the featured image
set_post_thumbnail( $post_id, $attachment_id );
}
$post_id = 456; // Replace with the ID of the post you want to modify
$image_url = 'https://example.com/image.jpg'; // The URL of the image you want to set as the featured image
$result = set_featured_image_from_url( $post_id, $image_url );
if ( is_wp_error( $result ) ) {
echo 'Error setting featured image: ' . $result->get_error_message();
} else {
echo 'Featured image set successfully from URL.';
}
Here, $post_id
is the ID of the post where you want to set the featured image, and $image_url
is the URL of the image you wish to use.
Things to Consider
- File Existence and Permissions: For the local file example, ensure the file exists and the script has permission to access it.
- Valid URLs: For the URL example, ensure the URL is valid and the image is accessible.
- Error Handling: Both functions include error handling to manage situations like file not found or download errors.
- Use in WordPress Environment: These functions need to be used within the WordPress environment. They can be added to your theme’s
functions.php
file or a custom plugin. - Server Configuration: Depending on your server configuration, you may need to adjust file paths or handle additional security considerations.
Conclusion
Rebuilding and reinserting images into the WordPress Media Library can be a straightforward process, whether you’re sourcing from a URL or an external directory. Choose the method that best suits your situation, always keeping in mind best practices for website management and data handling.
Remember, while manual methods work well for a few images, consider scripting solutions for larger-scale operations to save time and reduce the risk of errors.