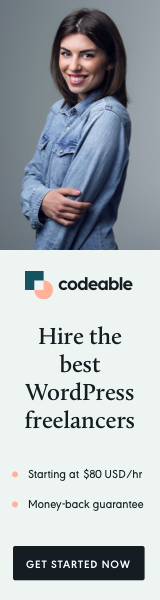
Keeping WordPress plugins up-to-date is generally a good practice, as updates often bring new features, improvements, and crucial security patches. However, there are instances where you might want to prevent a specific plugin from updating. This could be due to compatibility issues, custom modifications, or other reasons.
In this blog post, we’ll explore how to programmatically disable updates for a specific WordPress plugin. This method gives you more control over your site’s plugin management, especially when automatic updates aren’t suitable for your setup.
The Code Snippet
To disable updates for a specific plugin, you can use the following code snippet in your WordPress site:
function disable_plugin_updates( $value ) {
// Replace 'plugin-folder/plugin-file.php' with the actual path to the plugin's main file
$plugin_to_disable = 'plugin-folder/plugin-file.php';
if ( isset( $value ) && is_object( $value ) ) {
if ( isset( $value->response[$plugin_to_disable] ) ) {
unset( $value->response[$plugin_to_disable] );
}
}
return $value;
}
add_filter( 'site_transient_update_plugins', 'disable_plugin_updates' );
How It Works
- Locating the Plugin’s Main File: First, you need to find the main file of the plugin you wish to disable updates for. This is usually found in the plugin’s folder in the format ‘plugin-folder/plugin-file.php’.
- Adding the Function: Place the function in your theme’s
functions.php
file or in a custom site-specific plugin. This function hooks into WordPress’ssite_transient_update_plugins
filter, which is used to check for plugin updates. - How the Function Operates: The function checks the list of plugins due for updates. If it finds the specified plugin (
$plugin_to_disable
), it removes it from the update list. This effectively prevents WordPress from displaying update notifications for that plugin.
Things to Consider
- Compatibility Issues: Disabling updates can lead to security vulnerabilities and compatibility issues, especially if the plugin is crucial for your site’s functionality or security.
- Update Notifications: While this method stops updates, it doesn’t remove the plugin from the WordPress.org repository. The plugin will still be listed there, but your site won’t notify you of updates.
- Use With Caution: This approach should be used judiciously. Regular updates are important for the health and security of your WordPress site.
- Temporary Solution: Consider this a temporary measure. If you’re waiting for a compatibility fix or preparing your site for a major update, this method can be helpful.
Controlling plugin updates gives you greater management over your WordPress site, especially when dealing with custom configurations or compatibility issues. However, it’s essential to weigh the benefits against potential security risks. Always aim to keep your plugins updated whenever possible, and use this method responsibly to maintain the overall health and security of your site.
Remember, this approach is for advanced users comfortable with editing their site’s code. If you’re unsure, it’s always wise to consult with a professional.